This text exhibits construct a easy suggestion engine utilizing GNU Octave, a high-level interpreted language, primarily supposed for numerical computations, that’s principally appropriate with MATLAB. A suggestion engine is a program that recommends gadgets comparable to books and flicks for patrons, usually of a website online comparable to Amazon or Netflix, to buy. Advice engines ceaselessly use statistical and mathematical strategies to estimate what gadgets a buyer wish to purchase or would profit from buying.
From a purely enterprise perspective, one wish to maximize the revenue from a buyer, discounted for time (a greenback right this moment is value greater than a greenback subsequent yr), over the period that the client is a buyer of the enterprise. In a long run relationship with a buyer, this in all probability signifies that the client must be pleased with most purchases and most suggestions.
Advice engines are “scorching” proper now. There are lots of makes an attempt to use superior statistics and arithmetic to foretell what clients will purchase, what purchases will make clients glad and purchase once more, and what purchases ship essentially the most worth to clients. Information scientists are attempting to use a spread of strategies with fancy technical names comparable to principal element evaluation (PCA), neural networks, and assist vector machines (SVM) — amongst others — to predicting profitable purchases and personalizing suggestions for particular person clients based mostly on their acknowledged preferences, buying historical past, demographics and different elements.
This text presents a easy suggestion engine utilizing Pearson’s product second correlation coefficient, often known as the linear correlation coefficient. The engine makes use of the correlation coefficient to determine clients with related buying patterns, and presumably tastes, and recommends gadgets bought by one buyer to the opposite related buyer who has not bought these gadgets.
Fast Set up Directions
This text accommodates pattern code for a easy suggestion engine written in GNU Octave. There are 4 information: simulate_purchases.m, recommend_purchases.m, csvreadfix.m, and randi.m. The randi.m information implements the randi integer random technology perform for earlier variations of GNU Octave.
Obtain and set up GNU Octave 3.6.2 if potential. You probably have GNU Octave 3.6 or later, you’ll not want the randi.m file. The simulation and suggestion software program runs a lot quicker underneath GNU Octave 3.6.2 than GNU Octave 3.2.4 on the writer’s Home windows 7 laptop computer.
Obtain the three information simulate_purchases.m, recommend_purchases.m, and csvreadfix.m to a working listing (folder). It’s in all probability prudent to make use of a separate listing/folder for working the engine. Obtain randi.m if wanted.
Launch GNU Octave (3.6 if potential). Change present listing to your working listing with the downloaded information.
To run simulation and suggestion with default settings, merely enter:
octave-prompt> simulate_purchases; ... octave-prompt> recommend_purchases;
The determine under exhibits the working listing after working the simulation which creates the acquisition information information SIM_xxx that are in comma separated values (CSV) format.
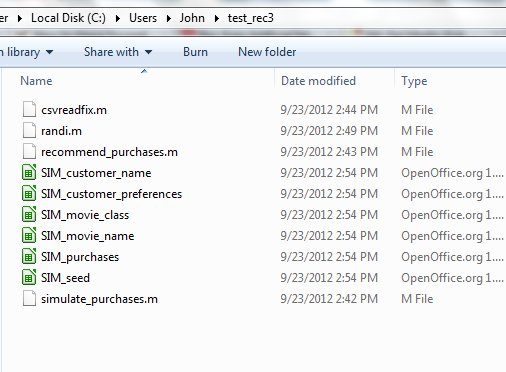
Working Listing with Advice Engine Information
Simulating Purchases
For illustration and growth functions, a simulator was developed that generates simulated buying information. The advice is for a hypothetical on-line video service that sells one-time views of flicks and different video for a small quantity, ninety-nine cents for instance. This straightforward suggestion engine doesn’t must know the value of the gadgets. The web video service has 4 classes of flicks: science-fiction, romantic comedy, motion/journey, and horror. An actual on-line video service would hopefully have a bigger choice of classes. The advice engine depends on the buying historical past and doesn’t must know the film classes.
The simulator and suggestion engine (see under) are GNU Octave features. These have been developed and examined on an HP laptop computer working Home windows 7 utilizing GNU Octave model 3.6.2. Notice that the simulation engine makes use of the randi perform which generates random integers uniformly distributed within the vary from 1 to N. randi is a brand new perform in Octave not present in earlier variations of Octave. An implementation of a randi perform for earlier variations of Octave could be discovered within the appendix. randi emulates a random quantity technology perform in MATLAB of the identical identify.
The simulator returns the simulated buying information in Octave “matrices” and saves the information to textual content information — comma separated values or CSV information with a file extension of CSV. The simulated information features a checklist of purchases — buyer CUSTOMER_ID purchased film MOVIE_ID, an inventory of simulated buyer names, an inventory of simulated film names, and the film class or class — film MOVIE_ID class CLASS_ID (science fiction, romantic comedy, motion, or horror).
simulate_purchases.m
perform [purchase_record, cust_names, cust_prefs, movie_name, movie_class] = simulate_purchases(ncust, npurchases, stem, debug) % [purchase_record, cust_names, cust_prefs, movie_name, movie_class] = simulate_purchases(ncust, npurchases, stem, debug) % % Inputs: % ncust -- variety of clients (default 100) % npurchases -- variety of purchases to simulate (default 100000) % stem -- stem for file names (default 'SIM' for simulated information) % debug -- debug hint flag (default=false) % % Outputs: % purchase_record -- document of purchases (buyer id, film id) % cust_names -- names of consumers % cust_prefs -- film class (scifi, romcom, motion, horror) preferences of consumers, 1-5 ranking % movie_names -- names of flicks % movie_class -- film class (scifi=1, romcom=2, motion=3, horror=4) % % (C) 2012 by John F. McGowan, Ph.D. % % simulate_purchases.m (A Simulator for a Advice Engine for an On Line Film Service) % % Simulates a sequence of on-line purchases of flicks by a gaggle of % clients. It then infers the preferences of the simulated % clients and recommends motion pictures they might like. % % That is an Octave script. % GNU Octave is a high-level interpreted language, primarily supposed % for numerical computations. It supplies capabilities for the % numerical answer of linear and nonlinear issues, and for % performing different numerical experiments. It additionally supplies intensive % graphics capabilities for information visualization and % manipulation. Octave is often used by way of its interactive % command line interface, nevertheless it will also be used to jot down % non-interactive applications. The Octave language is kind of much like % Matlab so that the majority applications are simply transportable. % % URL: https://www.gnu.org/software program/octave/ % % NOTE: to put in io and xlswrite in Octave 3.6.2 on Home windows 7 PC % % obtain and set up the io bundle home windows installer from SourceForge % Octave> pkg load io % Octave> savepath (defaults to .octaverc % % set seed for random quantity technology % this manner can generate the identical simulated information every time if wanted % VALIDATE THE ARGUMENTS TO THE FUNCTION if nargin 2 printf("processsing buy %d/%dn", buy, npurchases); fflush(stdout); mark = time(); finish % select a buyer purchaser = randi(ncust); purchasers = [purchasers purchaser]; % select film class for buy rating = cust_cdf(purchaser,finish)*rand(1, 1); idx = discover(cust_cdf(purchaser,:) > rating); class = idx(1); movies_purchased_class = [movies_purchased_class class]; index = randi(n(class)); movie_purchased = idxmat(class, index); movies_purchased = [movies_purchased movie_purchased]; customer_matrix(purchaser, movie_purchased)++; finish % loop over purchases % Buy Historical past Simulated disp('Writing Film Names to Information'); fflush(stdout); %xlswrite('movie_purchases.xls', 'movie_name', 'Name_Sheet'); purchase_record = [purchasers' movies_purchased']; if debug disp(sprintf('stem set to %sn', stem)); fflush(stdout); finish csvwrite([stem '_movie_class.csv'], movie_class); % film class csvwrite([stem '_customer_preferences.csv'], cust_prefs); % buyer prefernces for film courses (ranking 1-5 for every film class) csvwrite([stem '_movie_name.csv'], char(movie_name)); % names of the films csvwrite([stem '_customer_name.csv'], char(cust_names)); % names of the purchasers csvwrite([stem '_purchases.csv'], purchase_record); % (buyer id, film id) csvwrite([stem '_seed.csv'], myseed); % save the random quantity generator seed used to generate this buying information disp('ALL DONE'); finish % perform simulate_purchases
Recommending Purchases
As soon as there may be sufficient simulated or actual buying information, the advice engine makes use of Pearson’s product second correlation coefficient to determine clients with related shopping for patterns. For instance, John Smith and Phil Jones may buy solely science fiction motion pictures. On this case, their purchases could be extremely correlated. The engine recommends motion pictures that Phil Jones has bought however not John Smith to John Smith, and vice versa.
The advice engine works by constructing a buyer matrix with the client on one axis and the film on the opposite axis. The matrix entries are the variety of instances the client has purchased a selected film. Clients with related or equivalent tastes comparable to John Smith and Phil Jones are possible to purchase overlapping units of flicks. The engine computes the correlation coefficient for every pair of consumers from the client matrix. If the correlation coefficient is bigger than 0.5 and has a p-value, a measure of statistical significance, lower than 0.05 (statistician Ronald Fisher’s well-known arbitrary cutoff), then the engine makes a suggestion.
The p-value is theoretically the likelihood that the correlation is because of probability alone; there isn’t any true correlation within the outcomes. A p-value lower than 0.05 means there’s a lower than 5 % probablity that the correlation is because of probability alone. The p-value is de facto extra difficult than this easy rationalization and the issues with utilizing the p-value are mentioned in additional element within the earlier article Easy methods to Dangle Your self with Statistics.
A suggestion engine for motion pictures is just not a life or demise utility like constructing a bridge or a nuclear energy plant. We might nearly by no means besides a 5 % likelihood of error in a life or demise utility. However in an utility like recommending motion pictures, some errors are in all probability acceptable. Amazon and Netflix make many unsuccessful suggestions however clients hold coming again and shopping for. That is an utility the place it’s in all probability acceptable to make use of the p-value regardless of its identified limitations.
NOTE: The colon : is used closely in Octave (and MATLAB). WordPress shows the sequence colon right-parenthesis as smiley face :). The reader will see a couple of smiley-faces displayed within the supply code under. Merely choose, copy, and paste the code right into a textual content or code editor. It will appropriately paste the colon right-parenthesis sequence into the textual content or code editor — not a smiley face.
recommend_purchases.m
perform [purchase_recommendations] = recommend_purchases(stem, debug) % [purchase_recommendations] = recommend_purchases([stem, debug]) % % Inputs: % stem -- stem for file names of information with simulate or precise buying information (default="SIM") % debug -- debug hint flag (default=FALSE) % % Outputs: % purchase_recommendations -- really helpful purchases for patrons with sufficient information to make prediction % format of every row is [customer id movieid1 movieid2 ....] % % (C) 2012 by John F. McGowan, Ph.D. % % It is a GNU Octave script. % GNU Octave is a high-level interpreted language, primarily supposed % for numerical computations. It supplies capabilities for the % numerical answer of linear and nonlinear issues, and for % performing different numerical experiments. It additionally supplies intensive % graphics capabilities for information visualization and % manipulation. Octave is often used by way of its interactive % command line interface, nevertheless it will also be used to jot down % non-interactive applications. The Octave language is kind of much like % Matlab so that the majority applications are simply transportable. % % URL: https://www.gnu.org/software program/octave/ % % % if nargin = 360 mycor = corr(customer_matrix'); % columns are clients else % corrcoef is deprecated mycor = corrcoef(customer_matrix'); finish masks = eye(ncust); masks = ~masks; mycor = masks .* mycor; similars = zeros(ncust, 3); printf('discovering most related clients by correlationn'); fflush(stdout); mark = time(); for buyer = 1:ncust % progress message newtime = time(); if ((newtime - mark) > 1) printf('processing buyer %d / %dn', buyer, ncust); fflush(stdout); mark = time(); finish % discover a totally different buyer with essentially the most related shopping for patterns to the present buyer % mx = max(mycor(buyer, :)); % most correlation apart from self customer_index = discover(mycor(buyer, :) == mx); % % compute p-values test_result = cor_test(customer_matrix(buyer, :), customer_matrix(customer_index, :)); similars(buyer, 1) = customer_index; % the id of the opposite buyer similars(buyer, 2) = mx; % most correlation coefficient similars(buyer, 3) = test_result.pval; % p-value statistical significance for correlation coefficient if debug printf('%d: %d %f %fn', buyer, customer_index, mx, test_result.pval); fflush(stdout); finish finish % loop printf('carried out with computing correlations between customersn'); fflush(stdout); % ids of consumers with very related tastes % % default to Fisher's 0.05 p-value cutoff % customer_similar = discover(similars(:,2) > 0.5 & similars(:,3) 0); printf('instructed motion pictures for buyer %d %sn', buyer, cust_names(buyer,:) ); fflush(stdout); suggestions; purchase_recommendations(customer_idx, 1) = buyer; purchase_recommendations(customer_idx, 2:size(suggestions)+1) = suggestions; for okay=1:size(suggestions) printf('%sn', movie_name(suggestions(okay),:) ); fflush(stdout); finish % loop over suggestions finish % for loop disp('ALL DONE'); finish % perform recommend_purchases
The advice perform calls csvreadfix to learn among the information from the information information generated by the simulator.
csvreadfix.m
perform [strings] = csvreadfix(fname, debug) % [strings] = csvreadfix(fname, debug) % % Inputs: % fname -- file identify of file to learn % debug -- debug hint flag (default=FALSE) % % learn textual content traces from CSV file written utilizing GNU Octave csvwrite % % (C) 2012 by John F. McGowan, Ph.D. % if ~ischar(fname) error('FIRST ARGUMENT IS NOT A STRING -- SHOULD BE NAME OF A CSV DATA FILE (E.G. MYDATA.CSV) '); return; finish if nargin 1 string = string(1,:); finish if debug disp('debug'); disp('measurement(string)'); disp(measurement(string)); disp(string); fflush(stdout); pause(2); finish strings = [strings; string]; if debug disp(strings); fflush(stdout); finish line = fgets(fid); finish % whereas loop fclose(fid); finish % perform
Conclusion
This text exhibits construct a easy suggestion engine based mostly on Pearson’s product second correlation coefficient utilizing GNU Octave. It makes use of correlation coefficients to determine clients with related shopping for patterns and presumably tastes in motion pictures. There are lots of further methods to use correlations to buying information to make suggestions.
There are lots of extra superior statistical and mathematical strategies that may be utilized to suggestion engines. These embrace principal elements evaluation (PCA), clustering algorithms, neural networks, assist vector machines (SVM), and lots of different strategies from synthetic intelligence, sign processing, and mathematical modeling. Many of those extra superior strategies are associated to at least one one other and, in some instances, are literally the identical technique with a distinct identify or slight beauty variations.
© 2012 John F. McGowan
In regards to the Creator
John F. McGowan, Ph.D. solves issues utilizing arithmetic and mathematical software program, together with growing video compression and speech recognition applied sciences. He has intensive expertise growing software program in C, C++, Visible Fundamental, Mathematica, MATLAB, and lots of different programming languages. He’s in all probability greatest identified for his AVI Overview, an Web FAQ (Continuously Requested Questions) on the Microsoft AVI (Audio Video Interleave) file format. He has labored as a contractor at NASA Ames Analysis Middle concerned within the analysis and growth of picture and video processing algorithms and expertise. He has revealed articles on the origin and evolution of life, the exploration of Mars (anticipating the invention of methane on Mars), and low-cost entry to area. He has a Ph.D. in physics from the College of Illinois at Urbana-Champaign and a B.S. in physics from the California Institute of Know-how (Caltech). He could be reached at [email protected].
Appendix I: RANDI FUNCTION
The randi perform is new in current variations of GNU Octave. It isn’t current in earlier variations comparable to Octave 3.2. Under is the supply code for a GNU Octave randi perform for earlier variations of Octave. It’s in all probability higher to improve to model 3.6 of GNU Octave if potential. randi emulates a random quantity technology perform in MATLAB.
perform [result] = randi(imax, n, m, debug) % [result] = randi(picture [, n, m, debug]) % % (C) 2012 by John F. McGowan, Ph.D. if ~isnumeric(imax) error('FIRST ARGUMENT IS NOT A NUMBER -- SHOULD BE IMAX WHERE RANDI RETURNS INTEGER FROM 1 to IMAX'); return; finish if nargin